Object-Oriented Programming (OOP) is essential for any developer aiming to master the basics of structured and efficient programming. By adopting OOP, you learn to organize your code into objects, making it easier to manage complexity and improve code reusability. Whether you are a beginner or an experienced developer, this comprehensive guide will help you understand the fundamental principles of OOP and apply them to your development projects. Discover how to optimize your programming skills with OOP and transform your coding approach.
Summary:
- What is Object-Oriented Programming?
- History and Evolution of OOP
- Fundamental Concepts of OOP
- Advantages and Disadvantages of Object-Oriented Programming
- Object-Oriented Programming Languages
- Use Cases of OOP
- Best Practices in Object-Oriented Programming
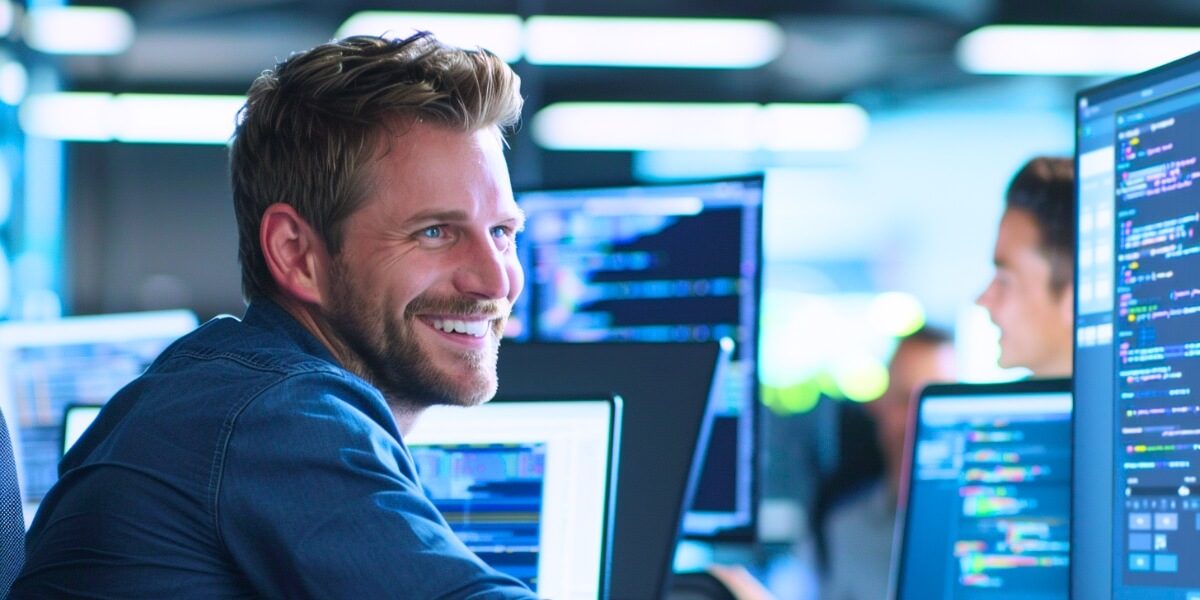
Key Points of the Article
- Object-oriented programming (OOP) is based on structuring code via classes and objects, making it easier to manage the complexity of modern software.
- The fundamental concepts of OOP, such as abstraction, encapsulation, inheritance, and polymorphism, are crucial for maintainable and scalable code architecture.
- Despite its advantages, OOP can pose challenges in terms of performance and complexity, requiring particular attention to memory management and code optimization.
What is Object-Oriented Programming?
Object-oriented programming is a programming model based on classes and objects. Unlike traditional paradigms that focus on logic and actions, OOP emphasizes objects and data. Each object has its own internal structure and behavior, allowing for better representation of real-world entities. This model aims to encapsulate data and associated behaviors, making code management easier.
One of the main goals of OOP is to translate the real world into code. By structuring code with classes to create instances of objects, developers can focus on the entities to be manipulated, which is particularly suited to complex programs.
Moreover, OOP offers considerable advantages such as code reusability, scalability, and efficiency. Object-oriented programming, or OOP, is a fundamental concept integrated into many modern programming languages.
History and Evolution of OOP
The history of object-oriented programming begins with Simula, recognized as the first object-oriented programming language. In the 1970s, Alan Kay pioneered OOP, defining its fundamental principles and influencing many languages that followed. In the 1990s, Java emerged as one of the most influential languages at the peak of OOP. Today, Java continues to dominate the object-oriented language market, thanks to its robust features and wide adoption in enterprise applications.
The evolution of OOP has been marked by increasing adoption of class and object concepts, facilitating the development of more modular and maintainable software. This transition to OOP has allowed for better management of the growing complexity of programs and has promoted collaboration among development teams through clearer code organization.
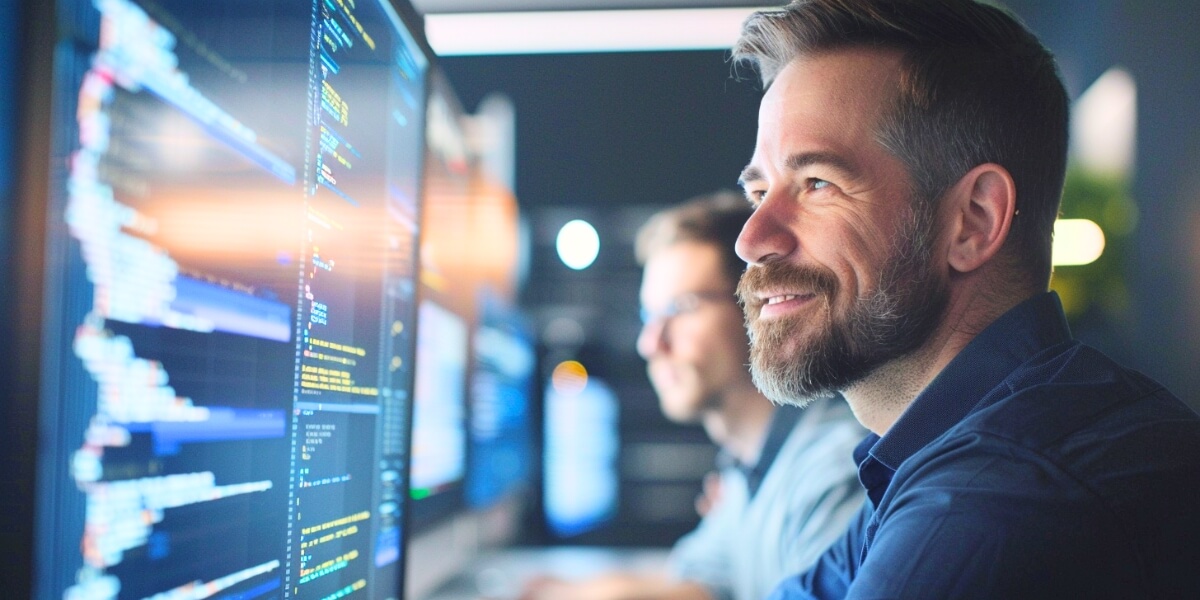
Fundamental Concepts of OOP
Object-oriented programming is based on five fundamental concepts that help structure software development:
- Abstraction
- Encapsulation
- Inheritance
- Polymorphism
These principles are essential for structuring code efficiently and facilitating its maintenance and evolution.
Let’s explore each of these concepts to understand how they contribute to the power of OOP.
Classes and Objects
Classes in object-oriented programming serve as templates from which objects can be created. They define the attributes and methods that derived objects will possess. For example, a “Car” class might define attributes such as color and model, as well as methods like start and stop.
Instantiation is the process by which an object is created from a class. This means using the template defined by the class to create a specific object with its own values for the attributes. For example, creating an object from the Car class could be represented by “my_car = Car()”.
An object in OOP is an instance of a class containing specific values for its attributes. Thus, instantiation brings the templates defined by classes to life, making OOP particularly powerful for modeling and manipulating real-world entities.
Encapsulation
Encapsulation is a central concept in object-oriented programming that involves hiding an object’s internal details and exposing only the necessary information. For example, a car shares public information through its lights but hides internal data under the hood. This protects data by preventing direct access and ensuring it can only be modified by the class’s specific methods.
This concept ensures controlled interaction with object properties, making code maintenance and security easier. By encapsulating data and behaviors, object-oriented languages allow these elements to be manipulated together coherently and securely.
Inheritance
Inheritance is a mechanism in object-oriented programming that allows for defining hierarchical relationships between classes to reuse attributes and methods. For example, a main “Employee” class can have a subclass “Manager” that inherits the characteristics of the main class while adding specific features in a journey.
This paradigm saves time and helps avoid code duplication by sharing common characteristics between classes. Inheritance also promotes a more organized and extensible code structure, facilitating the development and maintenance of applications.
Polymorphism
Polymorphism in OOP allows objects of different classes to use identical methods with varied behaviors. For example, a method “start()” can be defined in a “Vehicle” class and redefined in “Car,” “Motorcycle,” and “Boat” classes, illustrating polymorphism.
This concept, often associated with inheritance, allows handling objects of different types with a common interface. Using interfaces and method signatures, polymorphism enhances code flexibility and adaptability, making applications more robust and scalable.
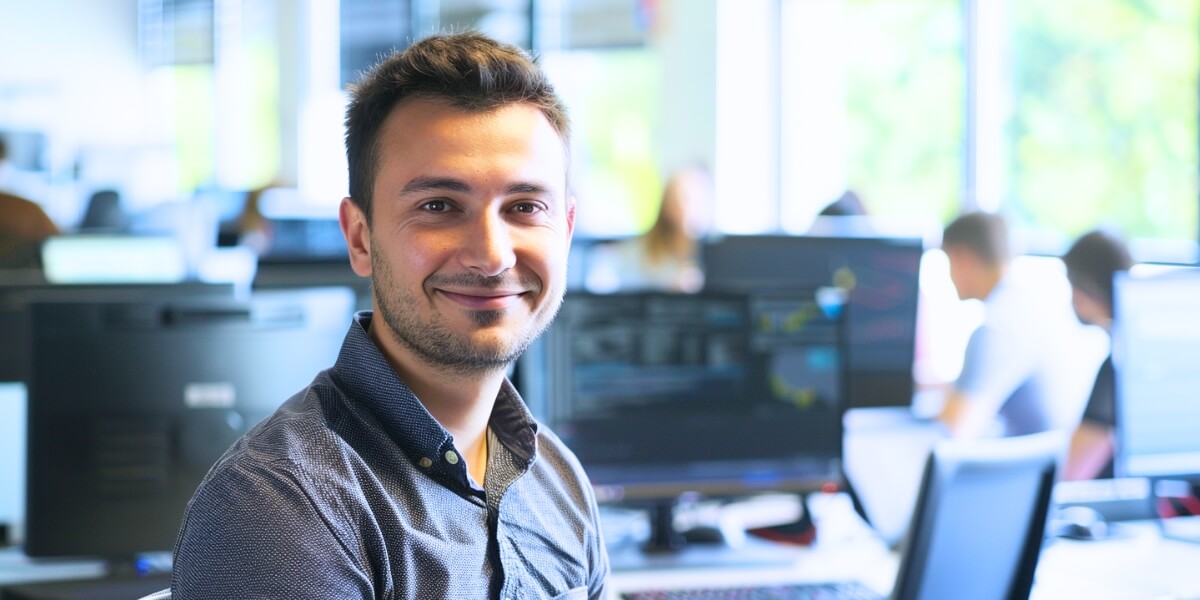
Advantages and Disadvantages of Object-Oriented Programming
Like any methodology, object-oriented programming has its advantages and disadvantages.
Let’s explore these aspects to better understand the impact of OOP on software development.
Advantages
Modularity is one of the main advantages of OOP, allowing independent code components to be created, easily integrated, and reused. An object class is reused in different programs, increasing development efficiency. In fields like data science, complex data is structured, and models are applied with reusable code thanks to the use of OOP.
OOP also facilitates program security by preventing unintended data modifications through encapsulation. In web development, it allows for creating more maintainable and collaborative code structures, thus improving application quality.
Using best practices, such as Test-Driven Development (TDD), significantly improves the quality of object-oriented applications.
Disadvantages
In summary, while OOP offers undeniable advantages, it is crucial to consider the challenges of complexity and performance in their evaluation. Manual memory management and performance optimization can add an extra layer of complexity to OOP-based projects.
Object-Oriented Programming Languages
Object-oriented programming languages include:
- Java
- Python
- C++
- C#
- and many others
Each of these languages offers unique features and specific advantages for object-oriented application development.
Let’s explore some of the most popular languages and how they implement OOP principles.
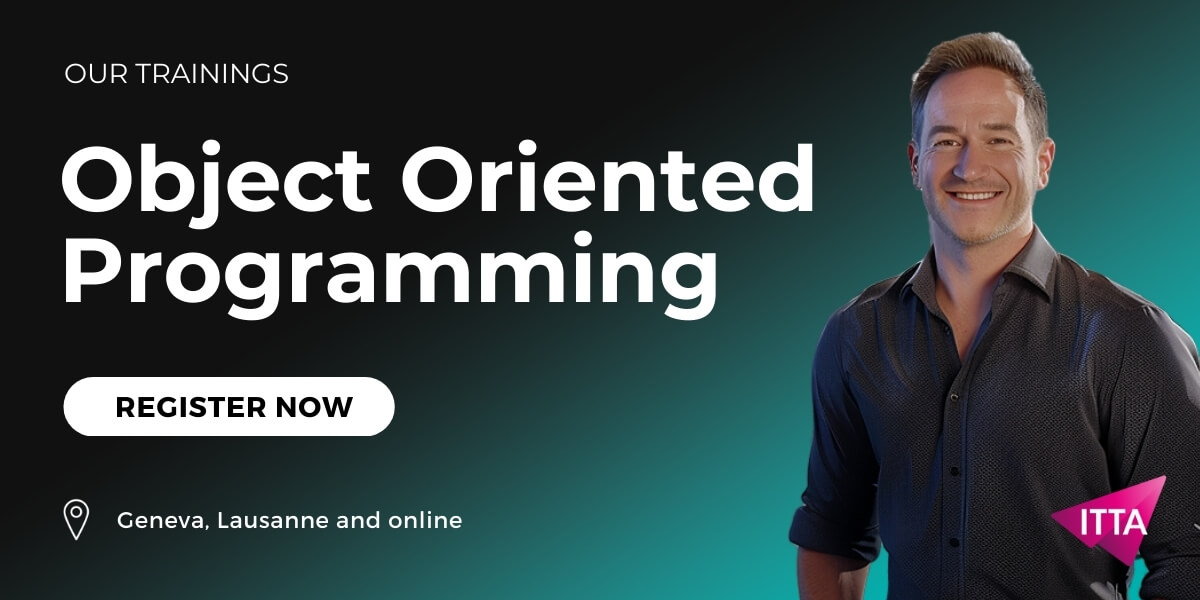
Java
Java is a robust and widely used programming language in enterprise applications. It uses object-oriented programming to model real-world interactions in the form of objects in code. The fundamental principles of OOP, such as encapsulation, inheritance, and polymorphism, are well integrated into Java, helping to create efficient applications.
Java enables the translation of real-world scenarios into logical and functional code structures, facilitating the development of complex software. Moreover, the language supports exception handling, allowing developers to manage errors that may occur during code execution.
The robustness of Java and its object-oriented features make it a preferred choice for many large companies, especially for developing information systems and large-scale applications.
Python
Python is a dynamic and easy-to-use programming language, ideal for beginners. It supports inheritance and polymorphism concepts, allowing flexible extension of class functionality. Python’s simple and intuitive syntax makes it an excellent choice for those who want to learn object-oriented programming without a steep learning curve.
Additionally, Python is widely used in various fields, including web development, data science, and automation, thanks to its ability to handle complex tasks with concise and readable code. This makes it a versatile and powerful language for a variety of applications.
C++
C++ is an object-oriented language offering advanced features, such as multiple inheritance and operator overloading, enhancing its capabilities. Memory management in C++ primarily uses dynamic allocation, giving developers precise control over the memory used by their programs.
C++ is known for its high performance, often superior to other object-oriented languages due to its proximity to hardware and explicit memory management. This makes it a preferred choice for applications requiring optimal performance and fine resource management.
C#
C# (pronounced “C Sharp”) is a modern, object-oriented programming language created by Microsoft for the .NET platform. It is designed to be simple, powerful, and secure, allowing for the development of a wide variety of applications, from desktop software to web and mobile applications. With its clear syntax and advanced features, C# is easy to learn and popular, especially in game development with Unity.
One of the major strengths of C# is its integration with the .NET platform, which offers a rich class library that simplifies many programming tasks. It also supports advanced concepts like asynchronous programming and automatic memory management while being compatible with multiple operating systems through .NET Core, making it a versatile and efficient choice for developers.
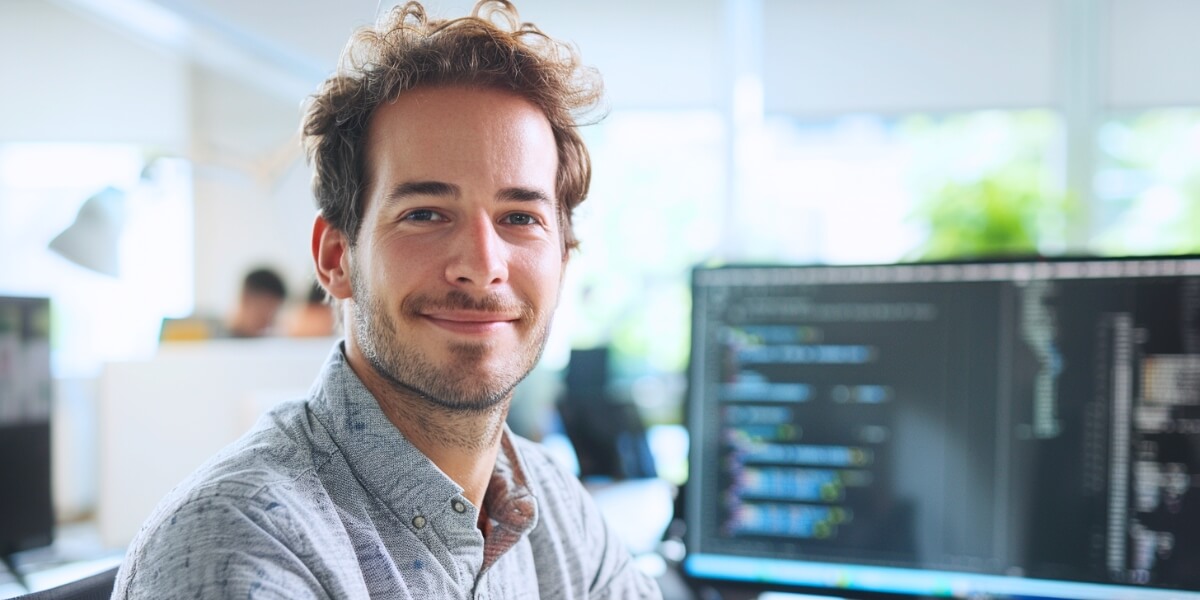
Use Cases of OOP
Object-oriented programming is often chosen for its ability to handle the increasing complexity of programs.
Let’s explore some use cases where OOP proves particularly effective.
Development of Complex Software
OOP is particularly suited for writing complex programs with difficult-to-manage data. By structuring the code into objects and classes, it facilitates complexity management and allows for better project organization. This is crucial in large-scale projects where many teams need to collaborate.
The modularity provided by OOP allows each team to work on specific components without interfering with other parts of the project. Using interfaces and abstract classes, OOP promotes code reuse in different contexts, which is a major asset for complex projects.
This also allows for defining clear contracts between different parts of the system, ensuring better integration and coherence of different functionalities.
Web Applications
In web applications, OOP structures code into objects, facilitating modularity and application maintenance. Many languages, such as Java, Python, and C++, support OOP and are commonly used for web development. OOP helps manage the complexity of modern web projects by promoting code reuse and making development more structured.
By facilitating code reuse and better organization, OOP enables the creation of robust and scalable web applications. This is essential in fast-paced development environments where quick code adaptation is necessary to meet user needs and technological changes.
Data Science
In data science, OOP facilitates processing large amounts of data by efficiently structuring the code. It offers advantages like modularity and reusability, which are essential for data science projects. OOP organizes complex data structures and algorithms into objects that interact smoothly.
The use of OOP is crucial for effectively structuring analytical projects, especially when data volumes are enormous. It allows data scientists to create reusable models and manage data pipelines more efficiently, ensuring more precise and faster data analysis.
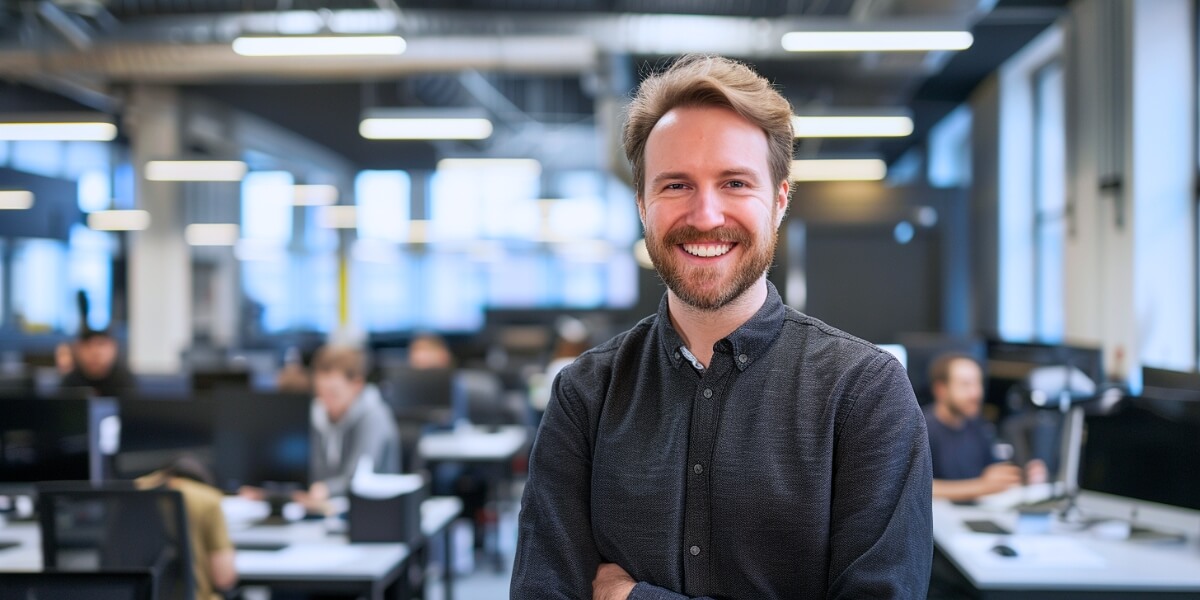
Best Practices in Object-Oriented Programming
To make the most of object-oriented programming, it is essential to follow some best practices.
These practices ensure a sustainable, flexible, and maintainable code architecture, contributing to the long-term success of software projects.
Designing Robust Classes
Strict adherence to OOP principles requires strict discipline in coding. Interfaces, which define method signatures that classes must implement, ensure code consistency and facilitate the design of robust and extensible classes.
Code Reusability
Code reusability is a fundamental principle of object-oriented programming that promotes application efficiency and maintainability. Applying composition rather than inheritance helps create independent and reusable modules.
This allows building modular systems that can easily be adapted or extended according to the changing needs of projects.
Unit Testing
Unit tests are essential in OOP because they verify that components work correctly and detect bugs early. By testing each class and method independently, developers ensure that each part of the code works as expected before its overall integration.
Unit tests help verify the behavior of classes in isolation and ensure that changes do not introduce regressions. By facilitating early bug detection, unit tests improve the overall quality of the code and reduce long-term maintenance costs.
Article Summary
In summary, object-oriented programming allows for efficient and maintainable code structuring. By using concepts such as classes, encapsulation, inheritance, and polymorphism, robust applications are created. For developing complex software, web applications, or data science projects, OOP is highly advantageous. By rigorously applying its principles and best practices, developers maximize the efficiency and quality of their projects.
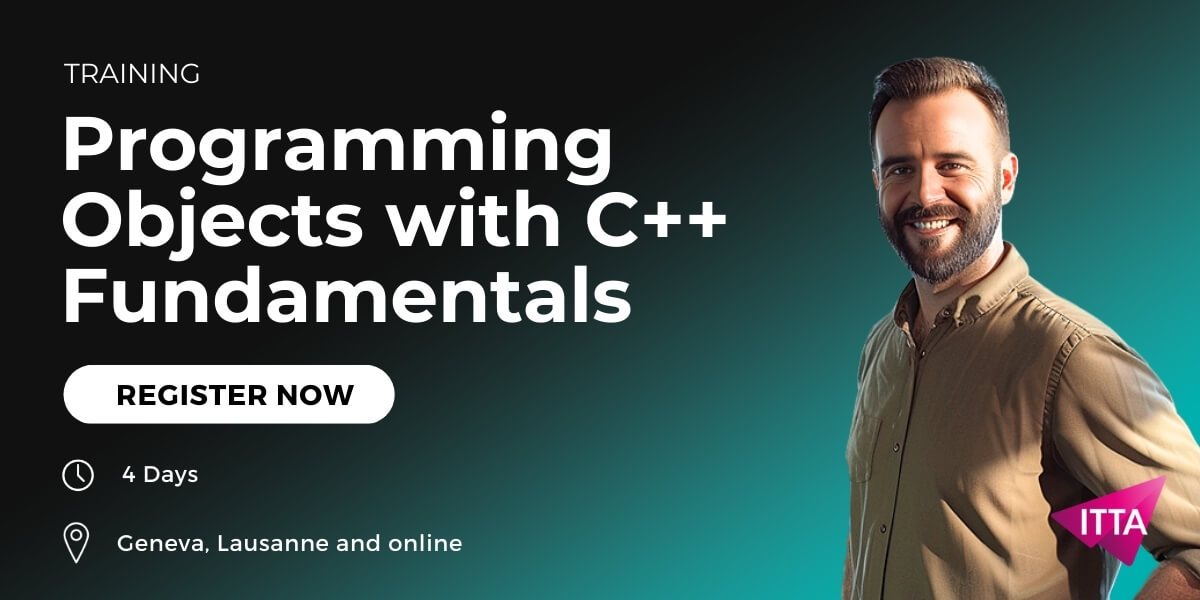
Frequently Asked Questions
What is Object-Oriented Programming?
Object-oriented programming is a programming model focused on classes and objects, emphasizing data management and objects rather than action logic. This paradigm allows structuring code in a modular and reusable way.
What are the fundamental concepts of OOP?
The fundamental concepts of object-oriented programming (OOP) include abstraction, encapsulation, inheritance, and polymorphism. These principles are essential for effectively structuring and organizing code.
What are the advantages of OOP?
Object-oriented programming (OOP) offers significant advantages such as modularity, code reusability, security, and ease of maintenance. These features make it a preferred choice for developing robust and scalable applications.
What are the disadvantages of OOP?
The disadvantages of object-oriented programming (OOP) include increased complexity and sometimes lower performance. Therefore, it is important to weigh these aspects when adopting this approach.
Which programming languages support OOP?
Object-oriented programming is supported by many languages, including Java, Python, C++, and JavaScript. You can choose one of these languages based on your specific needs.